BaseElementAdapter¶
The BaseElementAdapter class is a general element class that provides the access to the general data of an Allplan object such as name, common properties, geometry, … Objects of this class are e.g. returned as a result of an object selection.
Descriptive data¶
The data describing the object can be entered via the member functions
print("Name: ", base_element.GetDisplayName()) print("Drawing file number: ", base_element.GetDrawingfileNumber()) print("Element adapter type:", base_element.GetElementAdapterType().GetTypeName()) print("Model element UUID: ", base_element.GetModelElementUUID()) print("Is 3D element: ", base_element.Is3DElement())
The following output is shown
Name: Wall, straight Drawing file number: 1 Element adapter type: Wall_TypeUUID Model element UUID: bcdb12de-00fe-4ed8-b237-af6cb158adf3 Is 3D element: True
Common properties¶
The common properties are get by the function
print(base_element.GetCommonProperties())
with the result
CommonProperties( Color (7) Layer (3735) Stroke (1) Pen (1) ColorByLayer (0) PenByLayer (0) StrokeByLayer (0) HelpConstruction (0))
Geometry access¶
Four member functions can be used for geometry access, depending on the desired result. Starting from a wall with an opening, the result of the function call will be shown in the following sections.
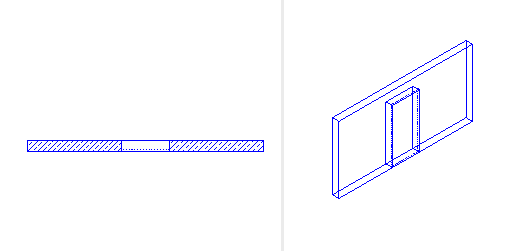
Attention: Since the wall consists of tiers, the geometry must be accessed via the wall tiers!
Selected geometry¶
The member function GetGeometry
print("Selected geometry:\n") print(base_element.GetGeometry())
returns the selected base geometry object, e.g. in case of object selection by the cursor. If a base object like line, spline, cylinder, … is selected, the geometry of the object is returned.
In case of architecture objects the result depends on the projection of the view used for the selection. A wall selection in the ground view e.g. returns the representation polygon of the selected wall like
Selected geometry: Polygon2D( Count(5) Points( (0, 0) (5000, 0) (5000, 240) (0, 240) (0, 0)))
If the wall is selected in an isometric view, the geometry of the 3D wall body is returned
Selected geometry: Polyhedron3D( Type(3) IsNegative(0) PartsCount(1) EdgeCount(12) FaceCount(6) VertexCount(8) Vertices( (0, 0, 0) (5000, 0, 0) (5000, 240, 0) (0, 240, 0) (0, 0, 2500) (5000, 0, 2500) (5000, 240, 2500) (0, 240, 2500)))
Model geometry¶
Access to the model geometry is provided by the member function GetModelGeometry
print("Selected geometry:\n") print(base_element.GetModelGeometry())
The function returns the 2D/3D geometry of the object as it is represented in the Allplan object model, including the cut-out of openings, …. In case of a wall with an opening the result looks like
Model geometry: Polyhedron3D( Type(3) IsNegative(0) PartsCount(1) EdgeCount(24) FaceCount(10) VertexCount(16) Vertices( (0, 240, 0) (0, 0, 0) (2000, 0, 0) (2000, 240, 0) (3010, 240, 0) (3010, 0, 0) (5000, 0, 0) (5000, 240, 0) (0, 0, 2500) (0, 240, 2500) (5000, 240, 2500) (5000, 0, 2500) (3010, 0, 2010) (2000, 0, 2010) (2000, 240, 2010) (3010, 240, 2010)))
Ground view architecture object geometry:¶
An architecture object has a special 2D geometry representation in the ground view, which can be accessed via the member function GroundViewArchEleGeometry
print("Ground view architecture object geometry:\n") print(base_element.GroundViewArchEleGeometry())
In case of a wall the result looks like
Model geometry: Polygon2D( Count(5) Points( (0, 0) (5000, 0) (5000, 240) (0, 240) (0, 0)))
Pure architecture object geometry:¶
If the pure geometry of an architecture object, e. g. without the openings, … is needed, the member function GetPureArchitectureElementGeometry can be used
print("Pure architecture object geometry:\n") print(base_element.GetPureArchitectureElementGeometry())
In case of a wall the result looks like
Model geometry: Polyhedron3D( Type(3) IsNegative(0) PartsCount(1) EdgeCount(12) FaceCount(6) VertexCount(8) Vertices( (0, 0, 0) (5000, 0, 0) (5000, 240, 0) (0, 240, 0) (0, 0, 2500) (5000, 0, 2500) (5000, 240, 2500) (0, 240, 2500)))
Attributes¶
To get the attributes of the object, the member function GetAttributes must be used
print("Attributes:") for attribute in base_element.GetAttributes(AllplanBaseElements.eAttibuteReadState.ReadAll): print(" ", attribute[0], AllplanBaseElements.AttributeService.GetAttributeName(doc, attribute[0]), "=", attribute[1])
In case of a wall the result looks like
Attributes: 10 Allright_Comp_ID = 0019Wal0000000398 12 Component ID = 398 49 Status = New building 83 Code text = 120 Calculation mode = m² 209 Trade = Concreting work 214 Component# = 220 Length = 5.0 221 Thickness = 0.24 222 Height = 2.5 226 Net volume = 3.0 229 Area = 12.5 498 Object_name = Wall 508 Material = 683 Ifc ID = 2lHXKxsQH3WgilI_LmUCVc
Sources¶
The BaseElementAdapterList is the list object for the BaseElementAdapter.
The complete documentation of the members from the BaseElementAdapter and BaseElementAdapterList class can be found here:
An example of the use of the BaseElementAdapter class can be found in the files
…\etc\Examples\PythonParts\ModelObjectExamples\General\ShowObjectInformation.pyp…\etc\PythonPartsExampleScripts\ModelObjectExamples\General\ShowObjectInformation.py