Input coordinate value by handle¶
This example shows the usage of a handle to input coordinate values:
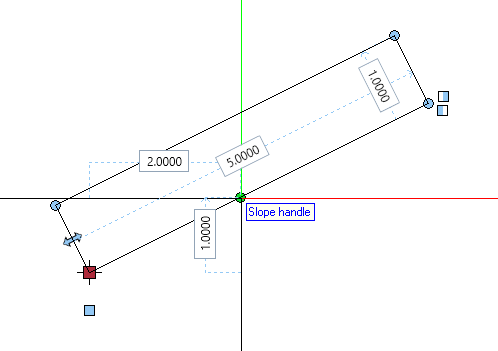
The Slope handle is used to input the values for the PythonPart parameters SlopeX and SlopeY. The source code for creating the handle looks like this:
handle_slope = HandleProperties("Slope",
point1 + AllplanGeo.Point3D(build_ele.SlopeX.value, build_ele.SlopeY.value, 0),
point1,
[HandleParameterData("SlopeX", HandleParameterType.X_DISTANCE, show_input_controls),
HandleParameterData("SlopeY", HandleParameterType.Y_DISTANCE, show_input_controls)],
HandleDirection.XYZ_DIR, False)
handle_slope.info_text = "Slope handle"
handle_list.append(handle_slope)
Special details¶
the handle parameter types X_DISTANCE and Y_DISTANCE are used to get the distance values in the xy-coordinate system, independent from a rotation of the PythonPart
the parameter abs_value is set to False. This allows to get also negative coordinate values for SlopeX and SlopeY.
the creation of the input fields is controlled by the value of show_input_controls.
Handle processing¶
The processing of the handle click and move can be done inside the PythonPart script as follows:
def move_handle(build_ele,
handle_prop: HandleProperties,
input_pnt: AllplanGeo.Point3D,
doc: AllplanElementAdapter.DocumentAdapter):
"""
Modify the element geometry by handles
Args:
build_ele: the building element.
handle_prop handle properties
input_pnt: input point
doc: input document
"""
build_ele.change_property(handle_prop, input_pnt)
return create_element(build_ele, doc)
Clicking and moving the handle shows a preview like this:
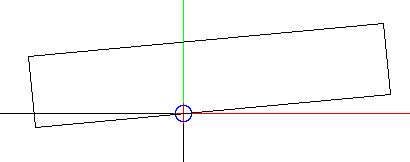