Input angle value by handle¶
This example shows the usage of a handle to input an angle value:
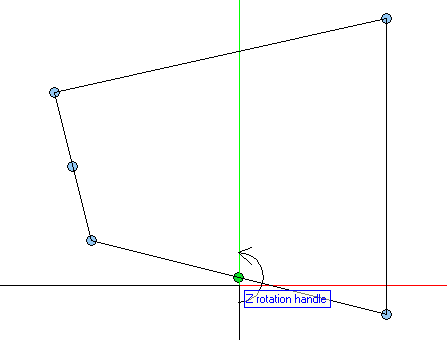
The Angle handle is used to input the value for the PythonPart parameter RotAngleZ. The source code for creating the handle for a rotation around the z-axis looks like this:
handle_z_rot = HandleProperties("Z_Rotation",
rot_pnt_z,
rot_pnt_z,
[HandleParameterData("RotAngleZ", HandleParameterType.ANGLE, show_input_controls)],
HandleDirection.ANGLE, False)
handle_z_rot.info_text = "Z rotation handle"
handle_list.append(handle_z_rot)
A free rotation axis can be defined by adding an angle placement like this:
handle_y_rot = HandleProperties("Y_Rotation",
rot_pnt_y,
rot_pnt_y,
[HandleParameterData("RotAngleY", HandleParameterType.ANGLE, show_input_controls)],
HandleDirection.ANGLE, False,
angle_placement = AllplanGeo.AxisPlacement3D(AllplanGeo.Point3D(),
AllplanGeo.Vector3D(1000, 0, 0),
AllplanGeo.Vector3D(0, -1000, 0)))
handle_y_rot.info_text = "Y rotation handle"
handle_list.append(handle_y_rot)
Special details¶
The modification of the handle is limited to the values of the following tags of the parameter
<MinValue> for the minimal value
<MaxValue> for the maximal value
<ValueList> for the allowed values
<IntervalValue> as grid length
Handle processing¶
The processing of the handle click and move can be done inside the PythonPart script as follows:
def move_handle(build_ele,
handle_prop: HandleProperties,
input_pnt: AllplanGeo.Point3D,
doc: AllplanElementAdapter.DocumentAdapter):
"""
Modify the element geometry by handles
Args:
build_ele: the building element.
handle_prop handle properties
input_pnt: input point
doc: input document
"""
build_ele.change_property(handle_prop, input_pnt)
return create_element(build_ele, doc)
Clicking and moving the handle shows a preview like this:
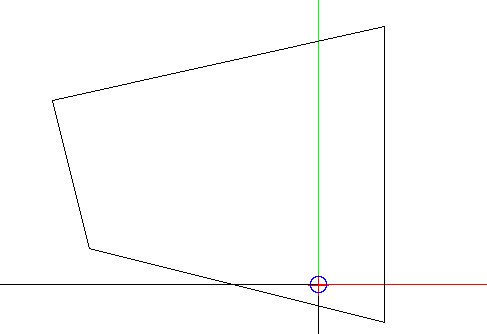