Button for incrementing or decrementing a value¶
This example shows the usage of a handle as increment or decrement button:
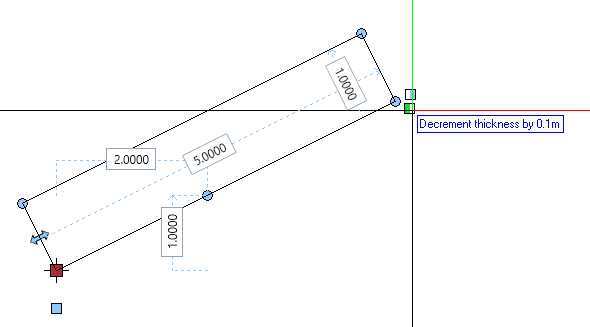
The DecrementHandle handle is used as button. The source code for creating the handle looks like this:
decrement_handle = HandleProperties("DecrementHandle",
point2 + AllplanGeo.Point3D(200, -100, 0), AllplanGeo.Point3D(),
[HandleParameterData("Thickness", HandleParameterType.DECREMENT_BUTTON,
in_decrement_value = 100.)],
HandleDirection.CLICK)
decrement_handle.info_text = "Decrement thickness by 0.1m"
decrement_handle.handle_angle = AllplanGeo.Angle(math.pi)
Special details¶
the handle parameter type DECREMENT_BUTTON is used to define the decrement button. INCREMENT_BUTTON can be used for the increment button.
each click to the button increments the value for Thickness by 100mm
the in_decrement_value is used to set the increment/decrement step
the handle is rotated by handle_angle
Handle processing¶
The processing of the handle click inside the PythonPart script can be done as follows:
def move_handle(build_ele,
handle_prop: HandleProperties,
input_pnt: AllplanGeo.Point3D,
doc: AllplanElementAdapter.DocumentAdapter):
"""
Modify the element geometry by handles
Args:
build_ele: the building element.
handle_prop handle properties
input_pnt: input point
doc: input document
"""
build_ele.change_property(handle_prop, input_pnt)
return create_element(build_ele, doc)
Clicking the handle shows a result like this:
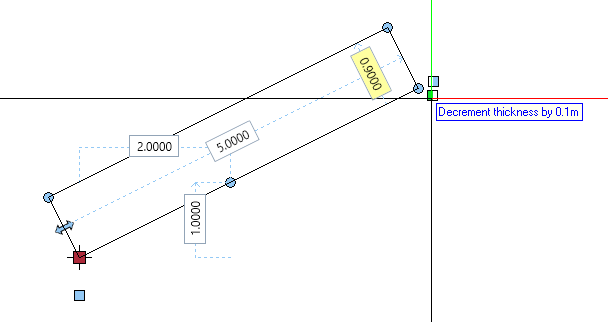